13届省赛pythonB组
[toc]
A 排列字母

我的题解
签到题
1 | import os |
正确题解
1 | string = 'WHERETHEREISAWILLTHEREISAWAY' |
B 寻找整数
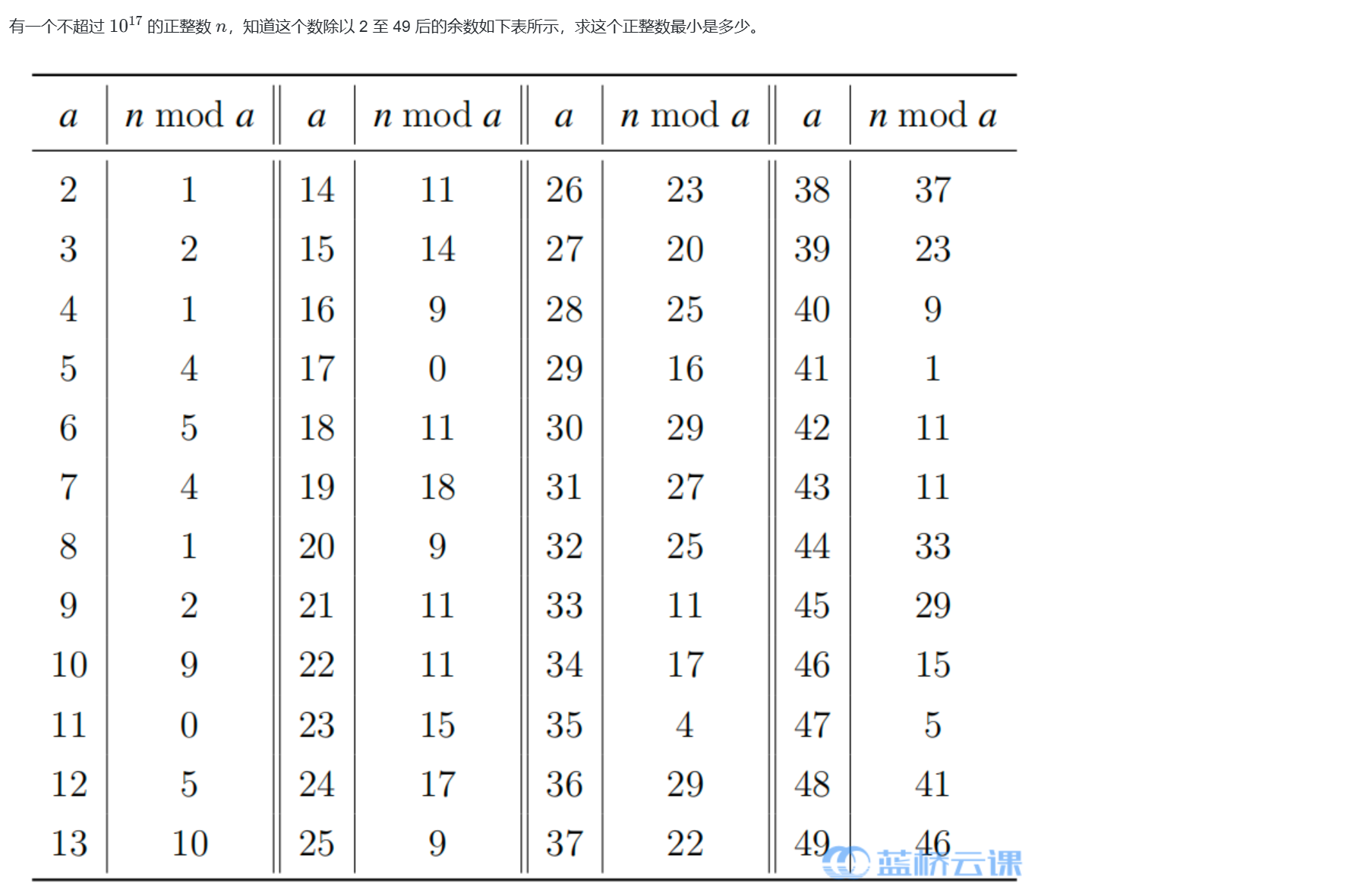
正确题解
参考题解0寻找整数 - 蓝桥云课
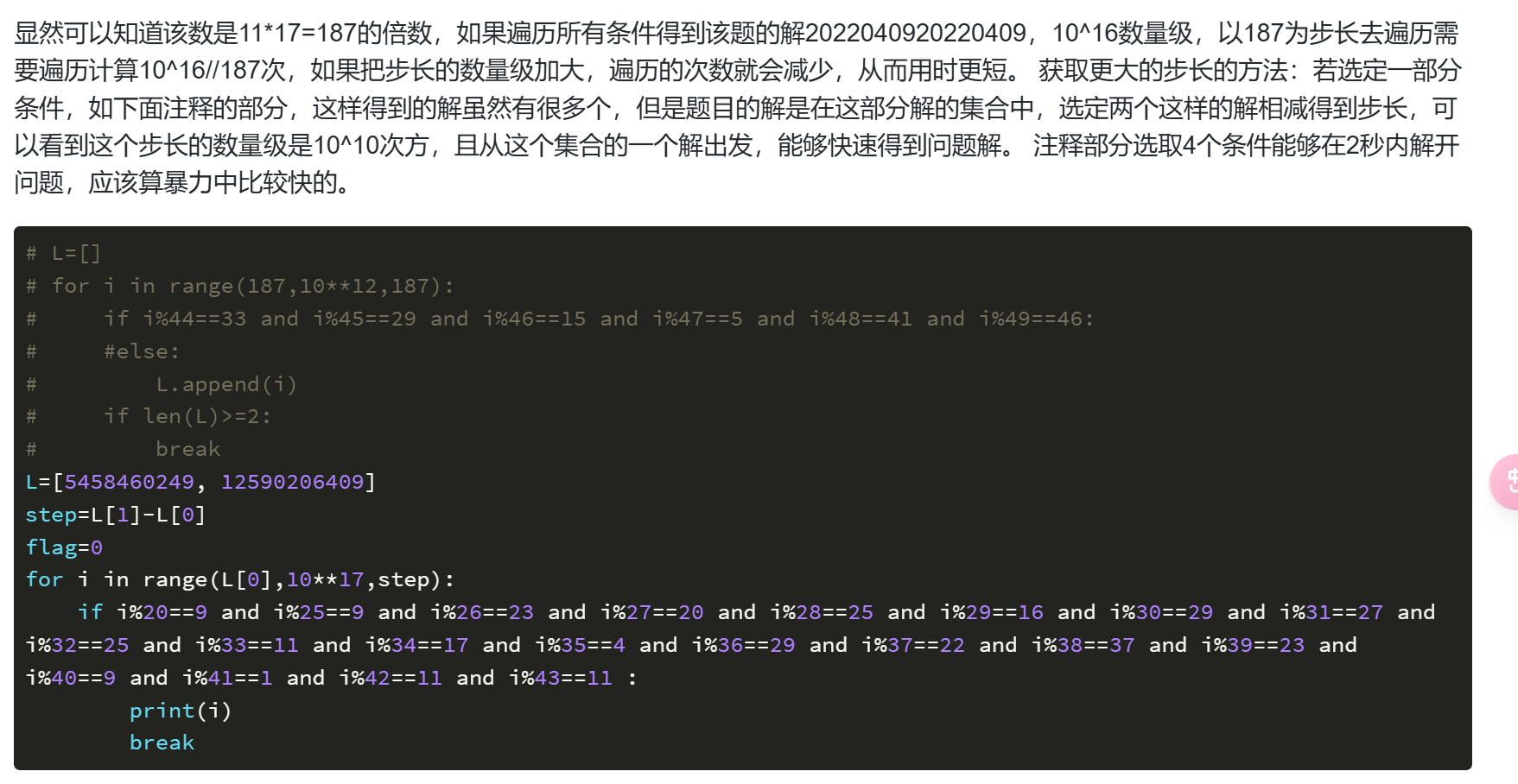
1 | L=[] |
C 纸张尺寸
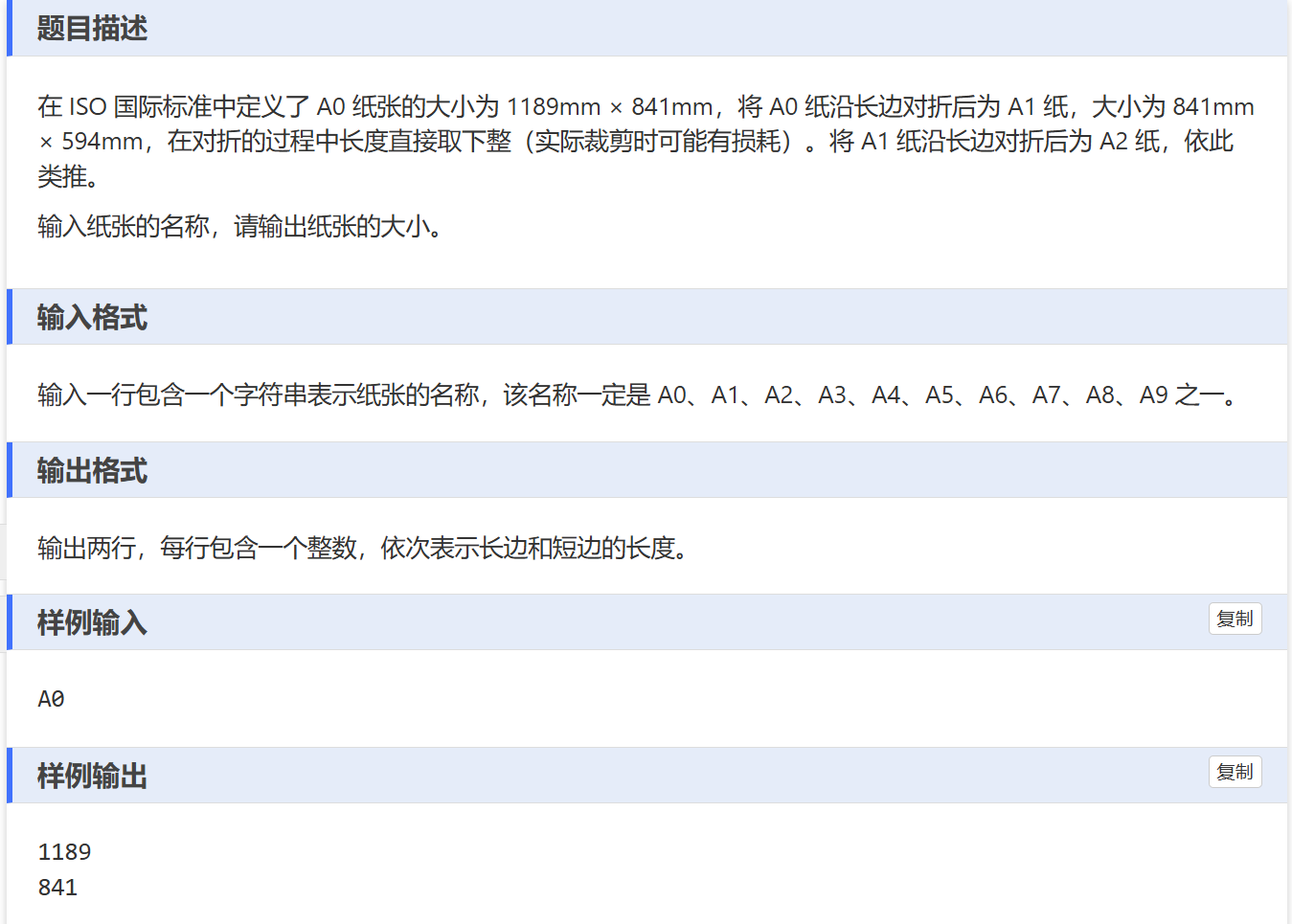
我的题解
1 |
|
D 数位排序
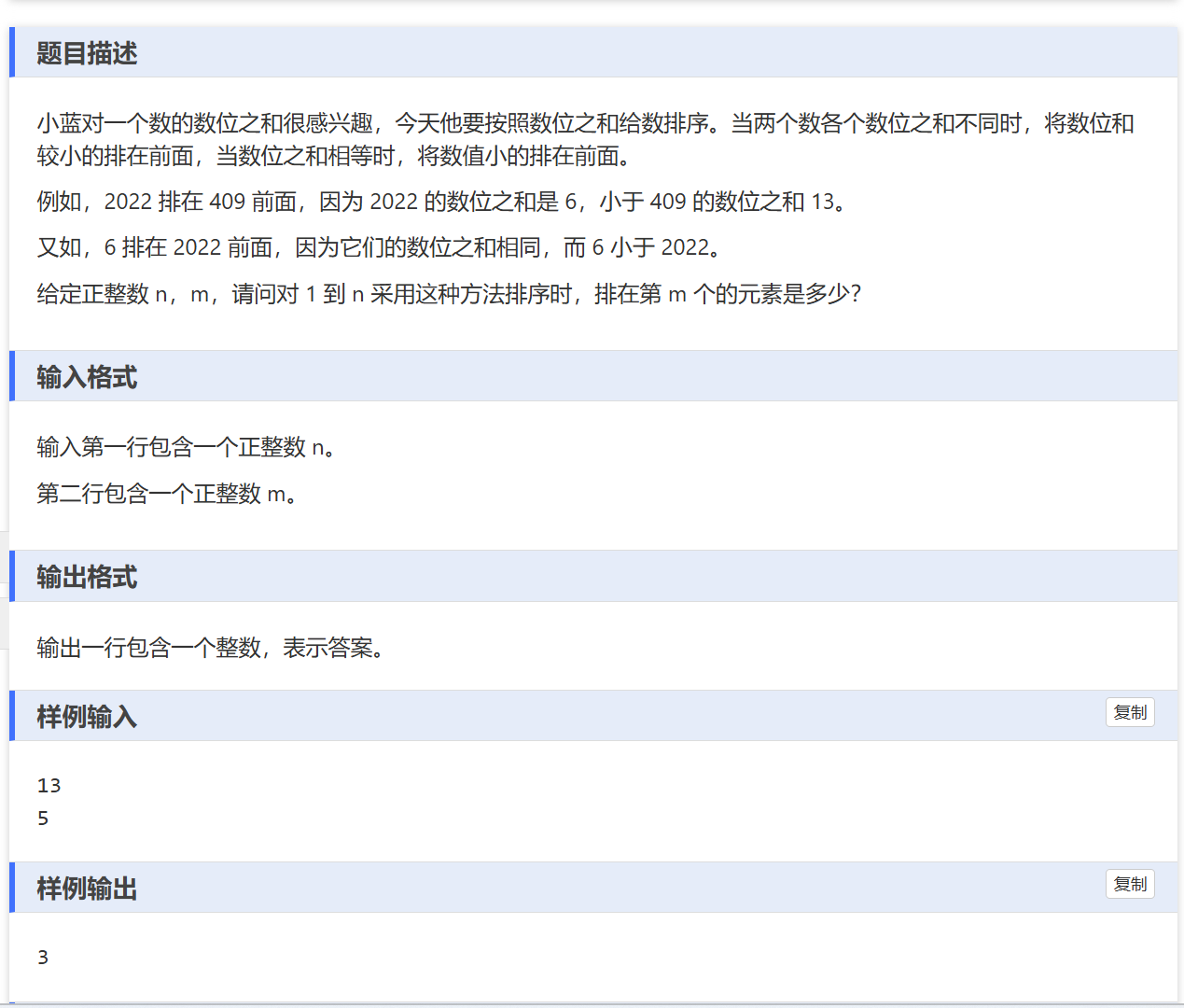
我的题解
1 | import os |
正确题解
1 | import os |
E 蜂巢
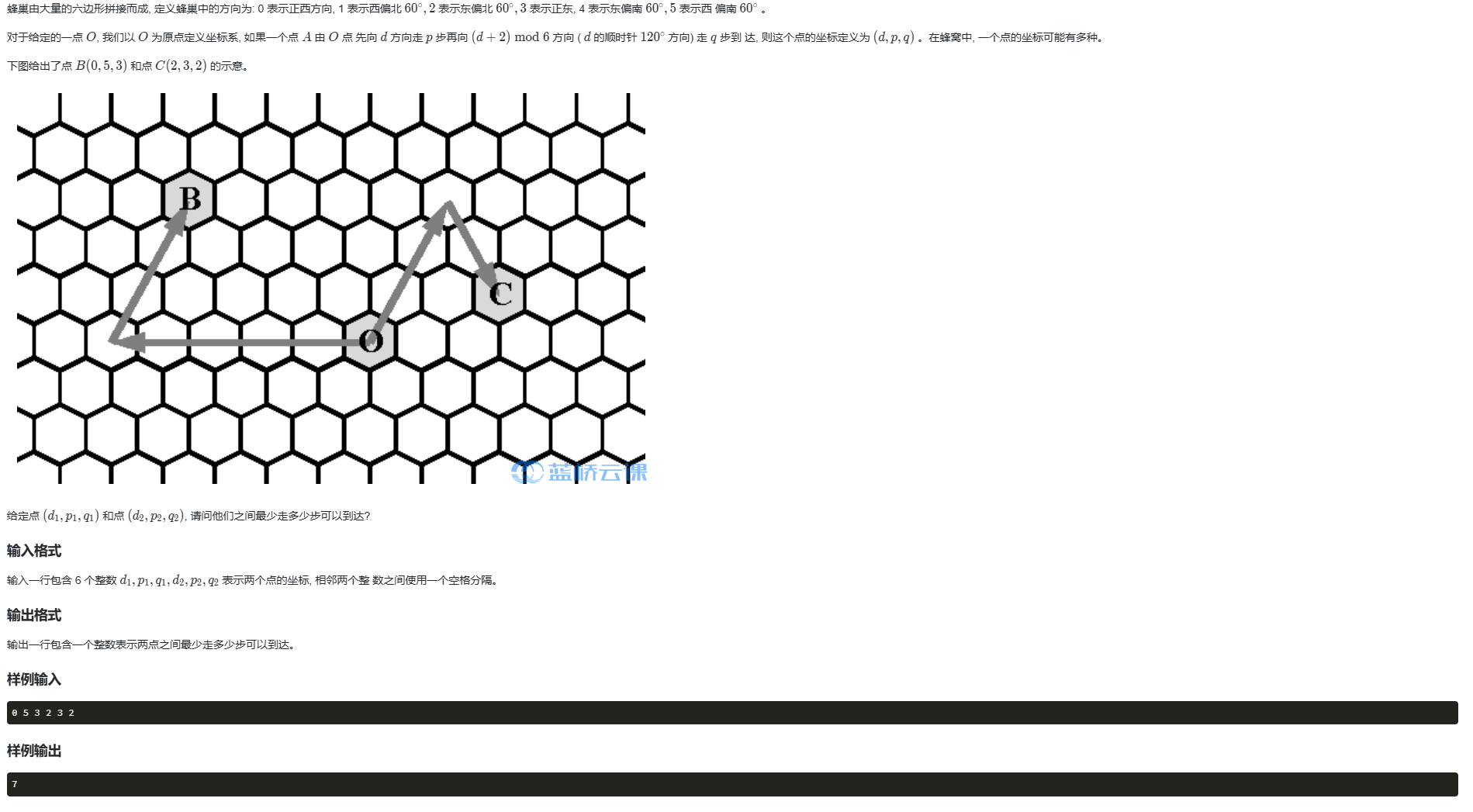
其实这题不是很难,要对自己有信心
正确题解
将六边形转化为直角坐标系,然后进行坐标计算
a到b点,考虑斜着走和先斜着走,在横着走的操作z
1 | import os |
F 消除游戏
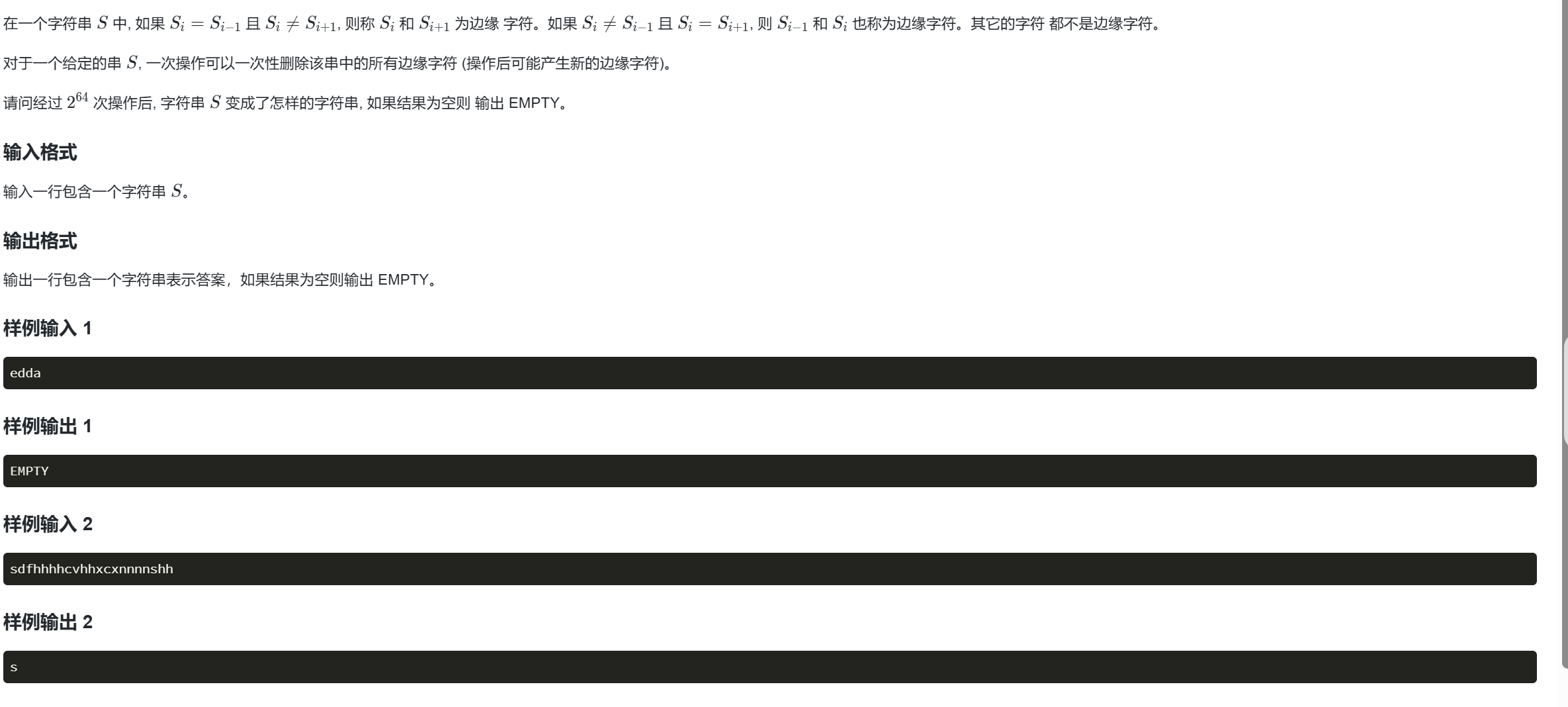
TODO
我的题解
1 | print("EMPTY") |
正确题解
G 全排列
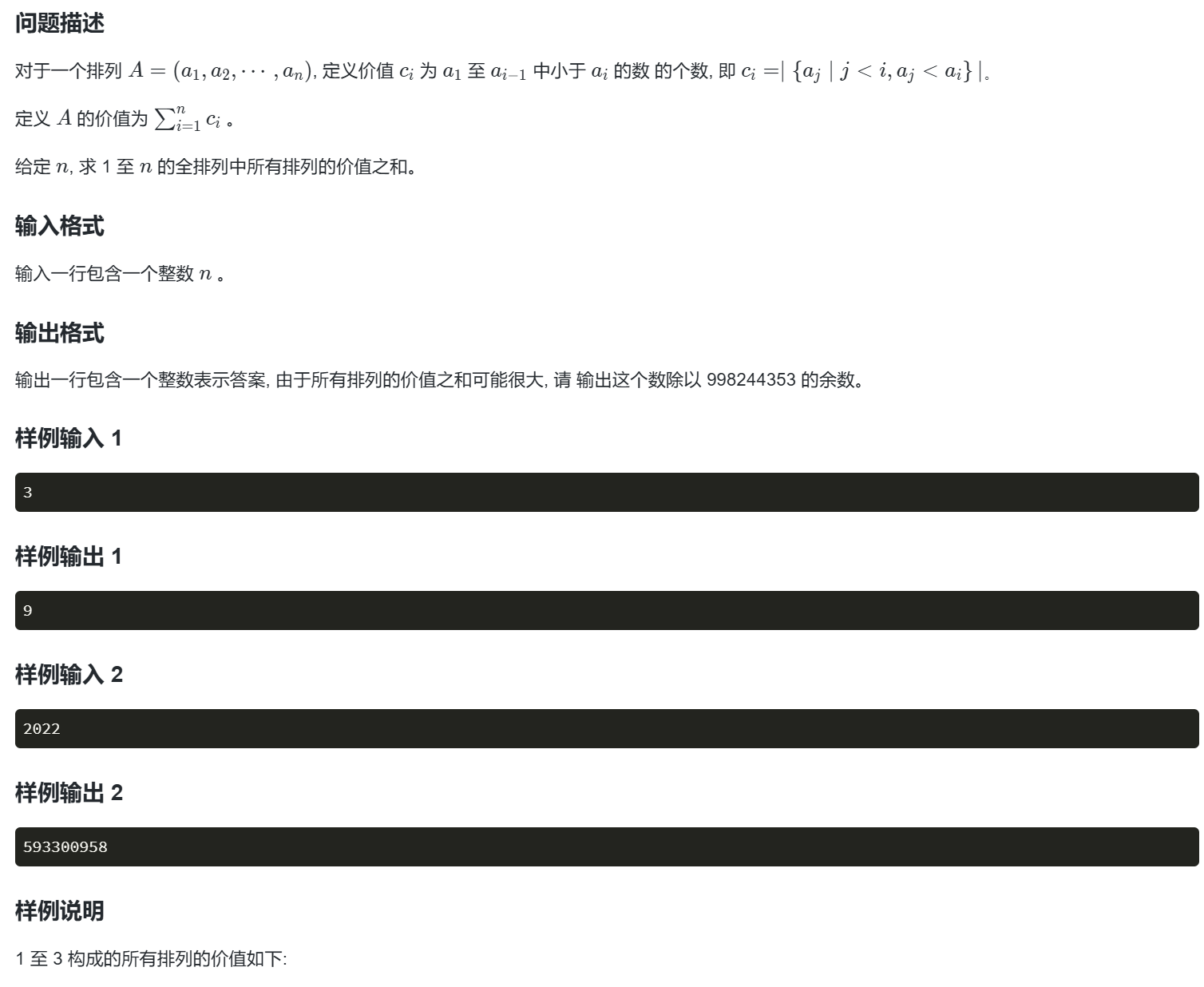
我的题解
想着dfs枚举推公式,然后推不出来
1 |
|
正确解法
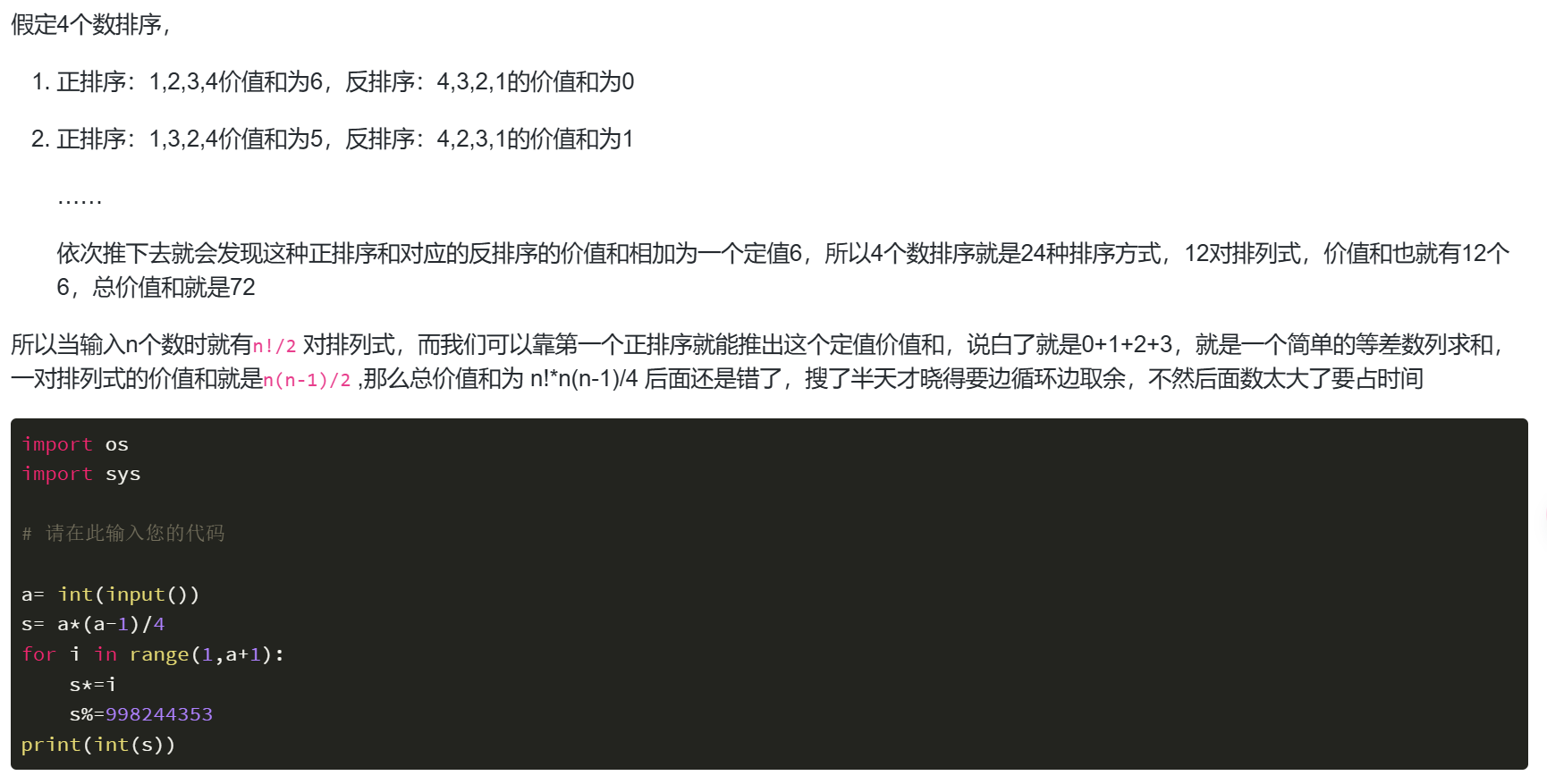
H
线段树,跳过
J 最优清零方案
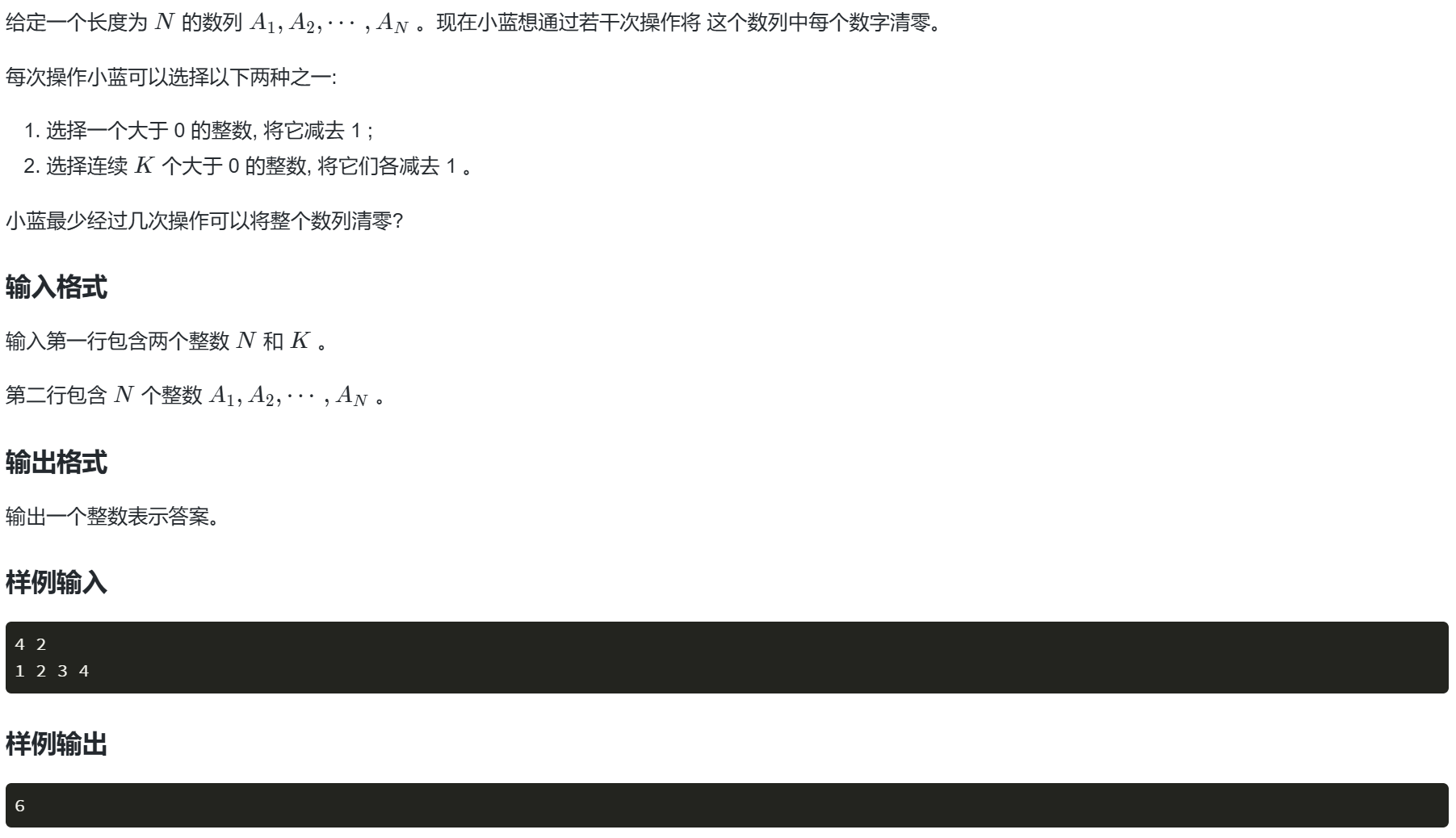
我的题解
通过枚举每个n//k个区间,减去最小值,加上剩余得单独处理的元素。
1 | import sys |
正确题解
果然还是不够贪啊
每次操作选择连续的
k
个元素,减去它们的最小值min_val
,操作次数加min_val
。- 剩余不足
k
的元素逐个减(操作次数加剩余元素的和)。
1 | import sys |
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来源 Randolfluo's blog!